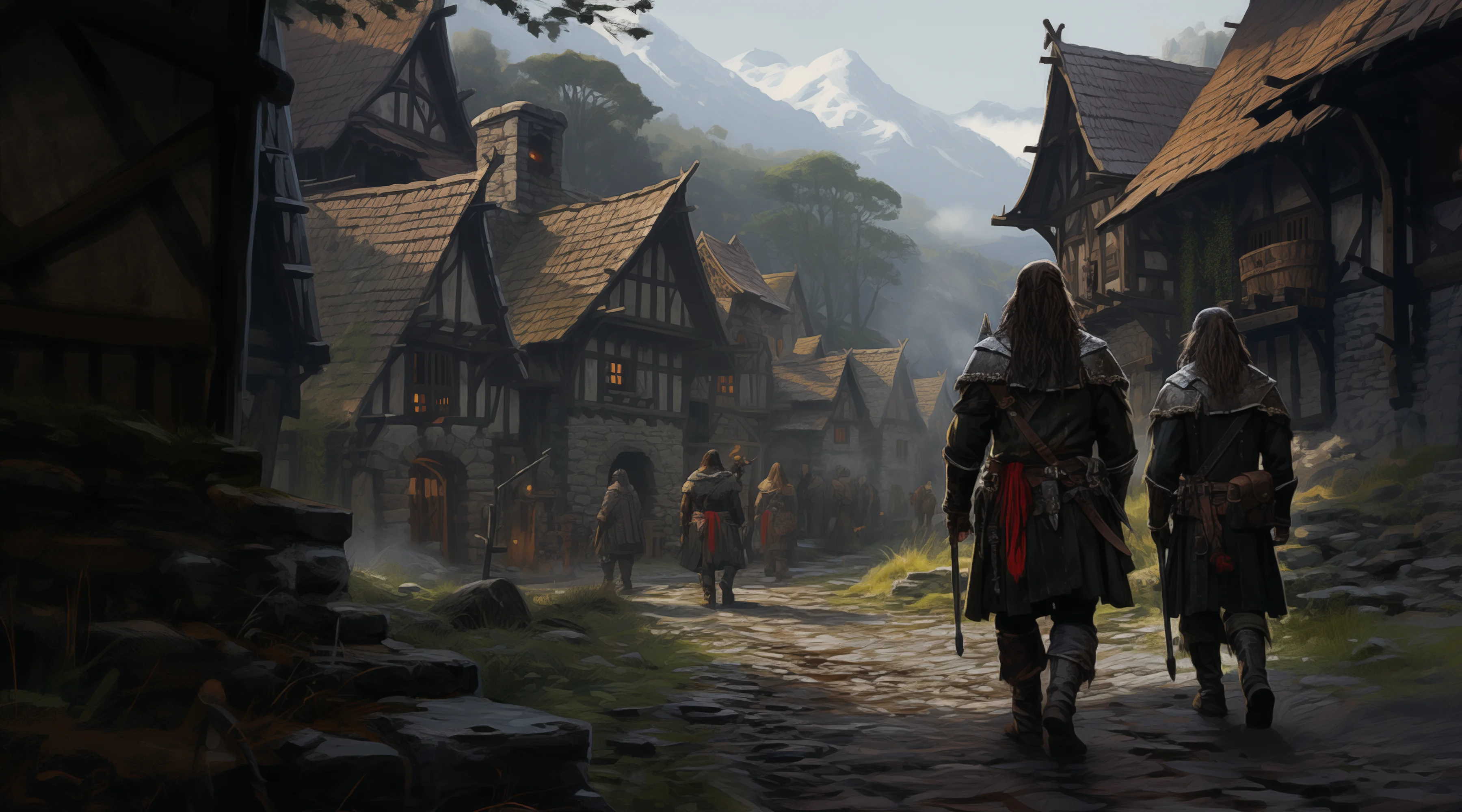
Standalone
Self-Hosted EVM
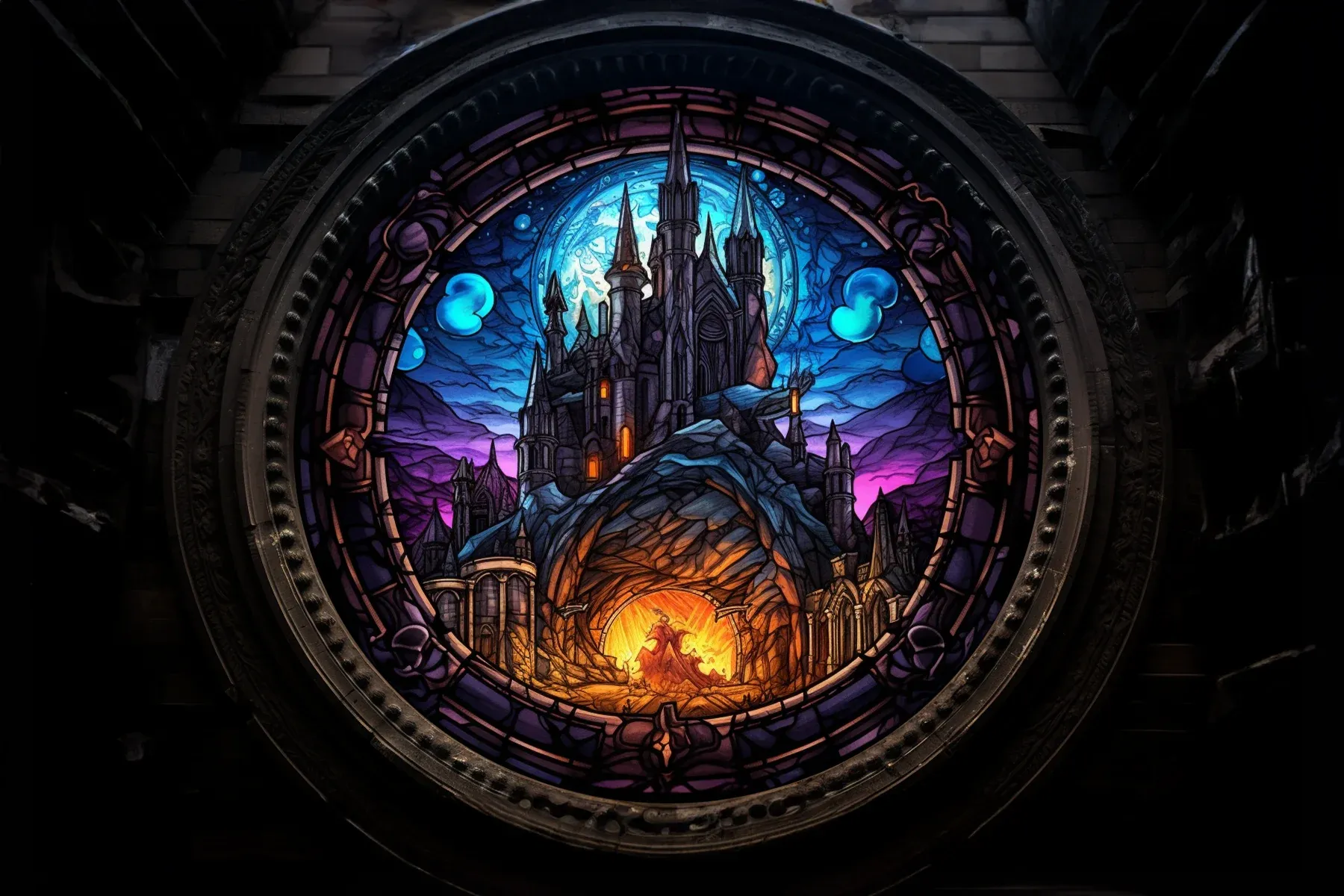
Part II
Stack
The EVM is a stack-based machine, where most interactions involve pushing and popping elements to and from a push-down stack. The stack holds elements, each 32 bytes long, and supports basic operations such as push and pop, and slightly more complex ones like peek and swap.
As we will see later, these primitives will lay the foundations of our solidity EVM and will be heavily used in most instructions' subroutines.
We will implement memory in contracts/libraries/Stack.sol
.
Push
Pushing elements onto the stack simply means adding the element to the top of the stack. This is the basic write operation for a stack.
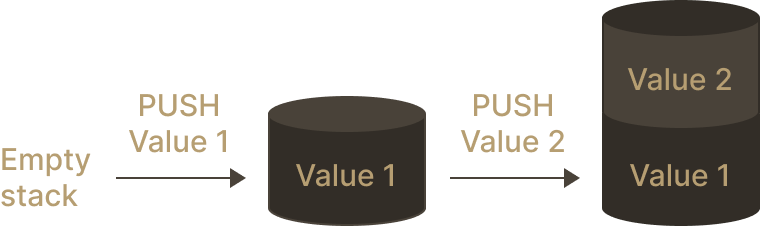
Pop / Peek
Popping reads and removes the top element from the stack.
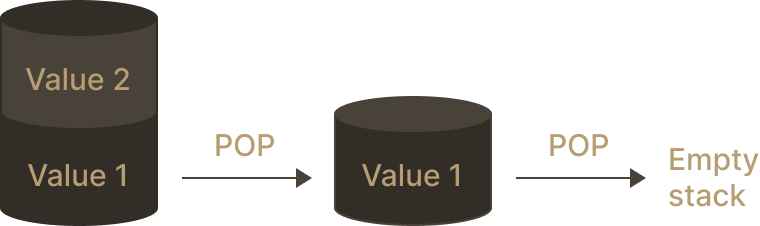
Peeking, on the other hand, merely reads an element in the stack. This will not modify the contents of the stack in any way.
Swap
Swapping elements will allow us to exchange the position of 2 elements in the stack. This should not modify the depth of the stack.
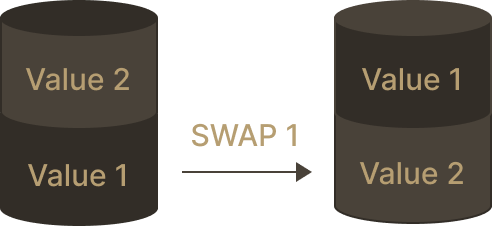
Function | Description |
| Initialize an empty stack with a maximum depth of |
| Push |
| Pop and return the topmost element from the stack |
| Return the element at the given depth in the stack |
| Swap the topmost element of the stack with the one at a specified |
Errors | Expected Message |
|
|
|
|
|
|
All required error messages are defined in contracts/libraries/Errors.sol
!
Your Task
Implement the functions in contracts/libraries/Stack.sol
.
Run tests in Questplay
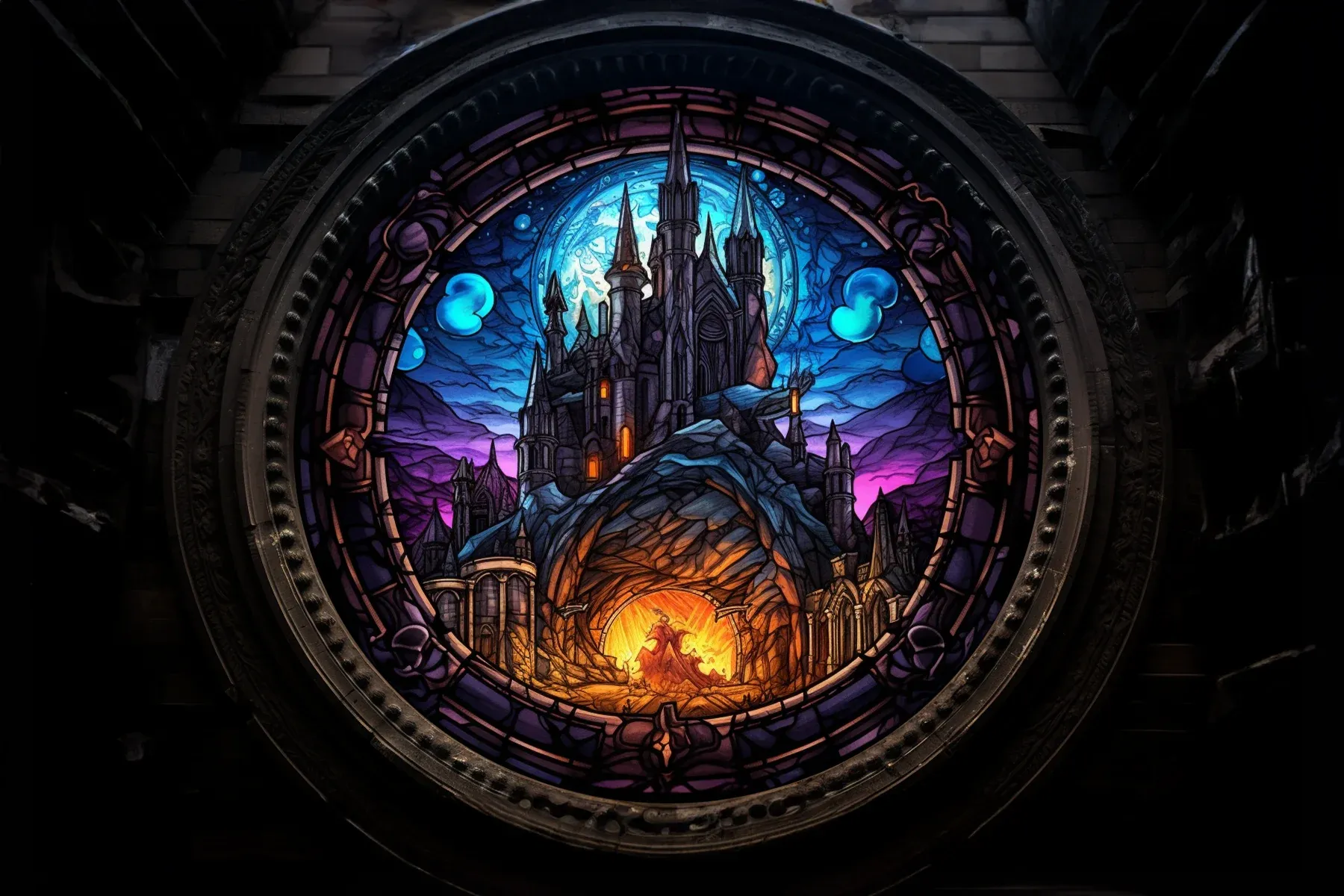
Over time, mages came together to form Guilds to learn to better understand the Weave. But corruption set in, and the Weaver Guilds sought to control the Weave for their own ends…