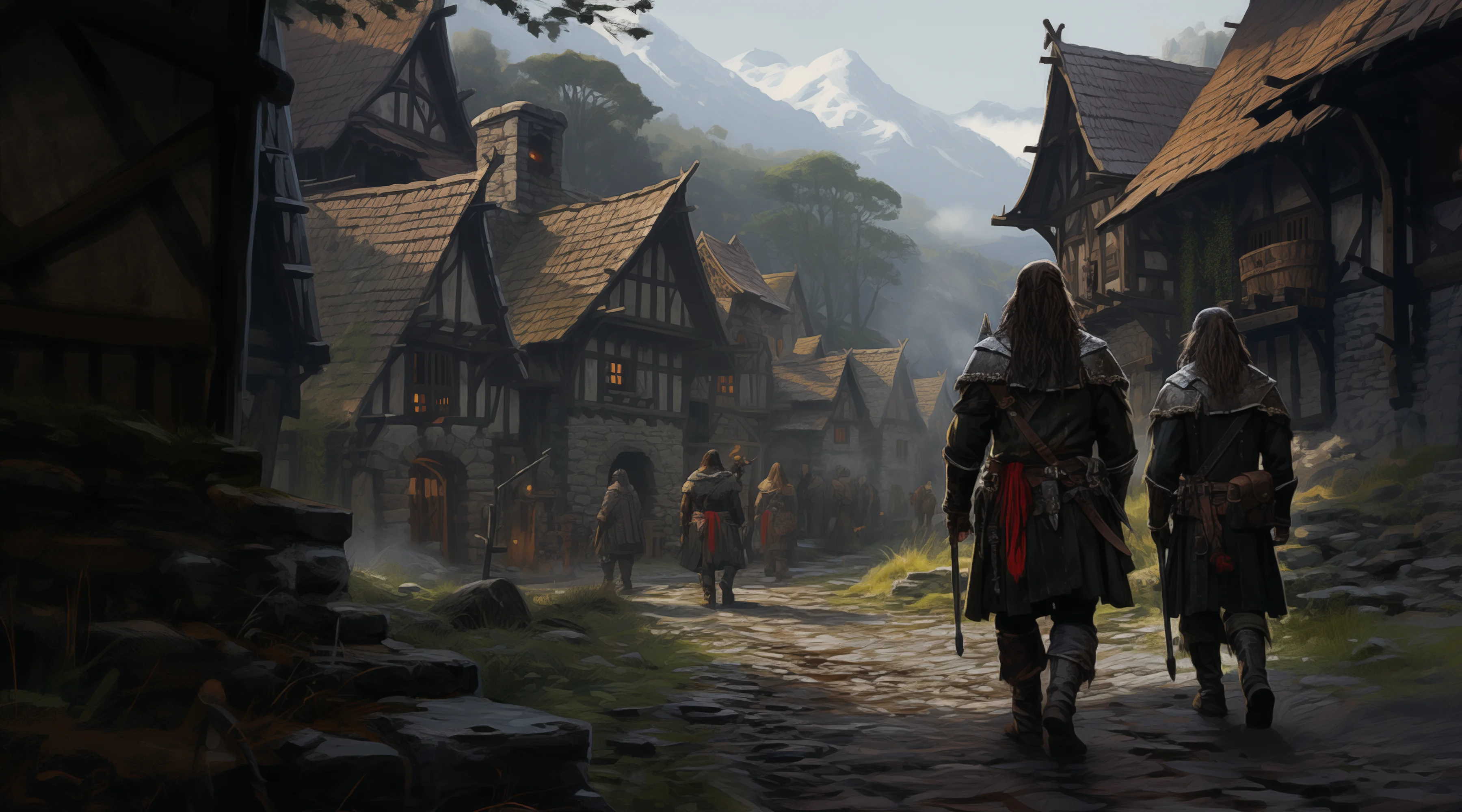
Standalone
Multicall
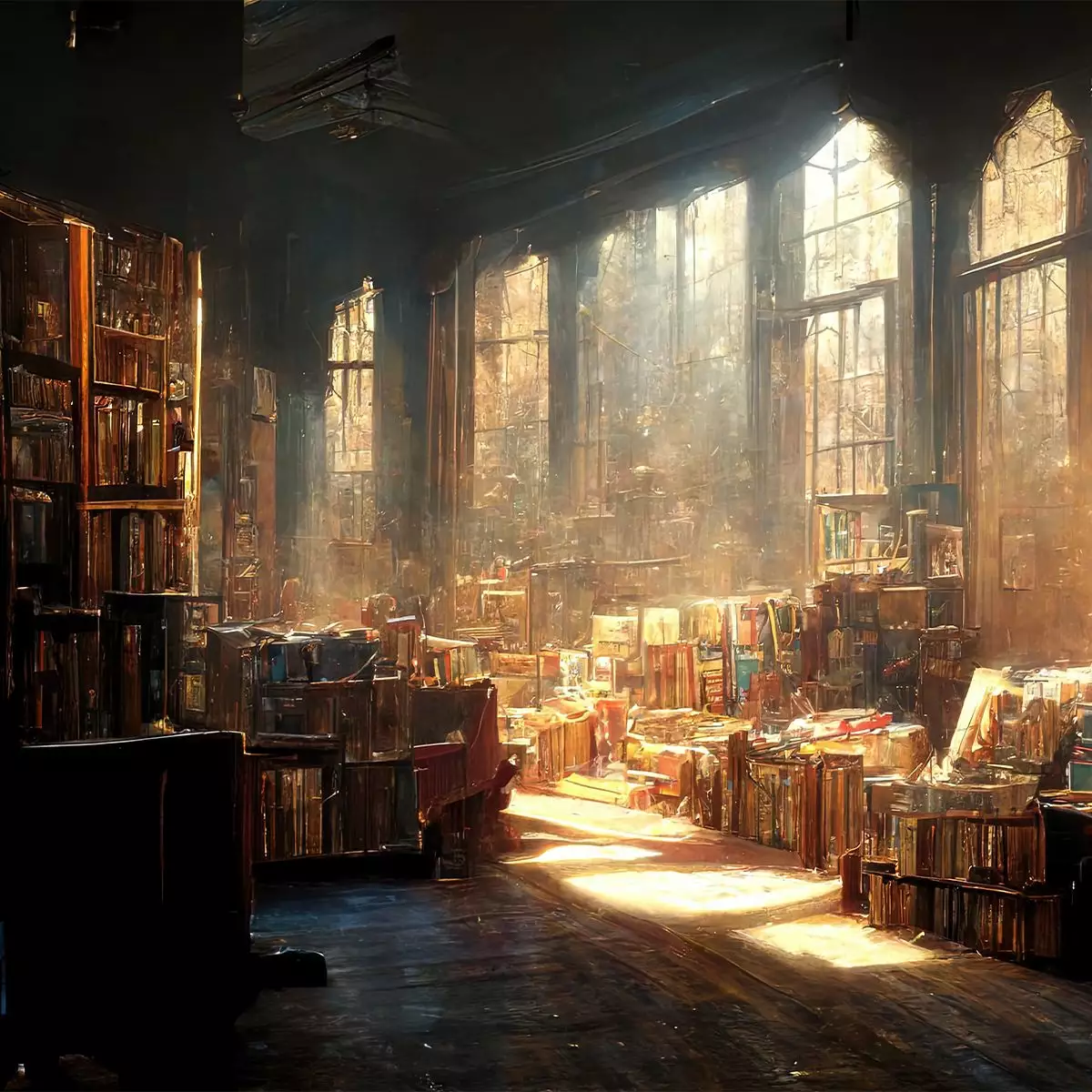
Part I
External Multicall
Download quests in Questplay
Take a look at the GreatArchives
contract. The scribes use this contract to store their records and scrolls. There are two ways of interacting with the contract's storage; reading and writing.
Read from contract | Write onto contract |
|
|
|
|
|
|
|
|
You do not have to concern yourself too much with the logic in GreatArchives
. Just know that it has several read and write functions on its storage.
The archives' scribes have to read many records and scrolls from the contract at a given time. Normally this will take numerous separate calls. Similarly, they have to write many records and scrolls onto the contract at a given time. This will take numerous individual transactions.
However, this might be too slow for the scribes to keep up with their substantial responsibilities. Ideally, the scribes should be able to perform multiple reads within one call, and multiple writes within one transaction.
Let us use a separate contract, GreatScribe
, to facilitate the desired batching of reads or writes (aka multicall).
For example, let us assume a scribe wishes to make the following read-only calls on GreatArchives
:
Record a = archive.historyRecords(21);
Record b = archive.magicRecords(11);
Record c = archive.historyRecords(31);
bytes d = archive.scienceScrolls(0xbA0c...);
The scribe wants to transform each of the four read-only calls into their respective ABI calldata and pass it to the GreatScribe
contract. GreatScribe
will then sequentially forward the calldata to GreatArchives
, and collect the four return values before sending it back to the scribe.
Your Task
Implement multiread()
and multiwrite()
in the GreatScribe
contract. The functions' specifications can be found in their NatSpec comments.
We want GreatScribe
to be able to work even if the code in GreatArchives
changes. In other words, GreatScribe
should not need any knowledge of the ABI in GreatArchives
to work.
Run tests in Questplay
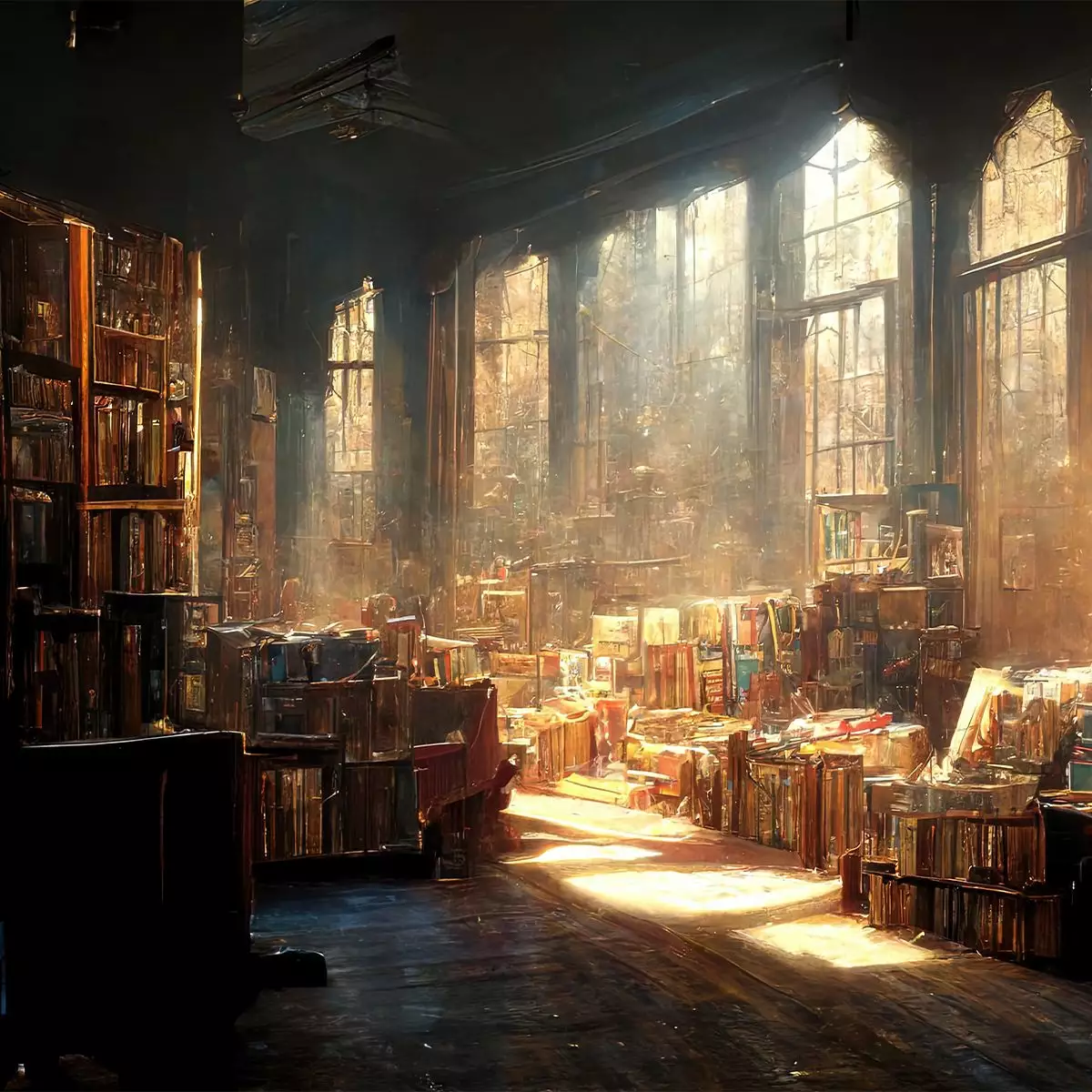
Help the scribes find an easier way to record their annals.