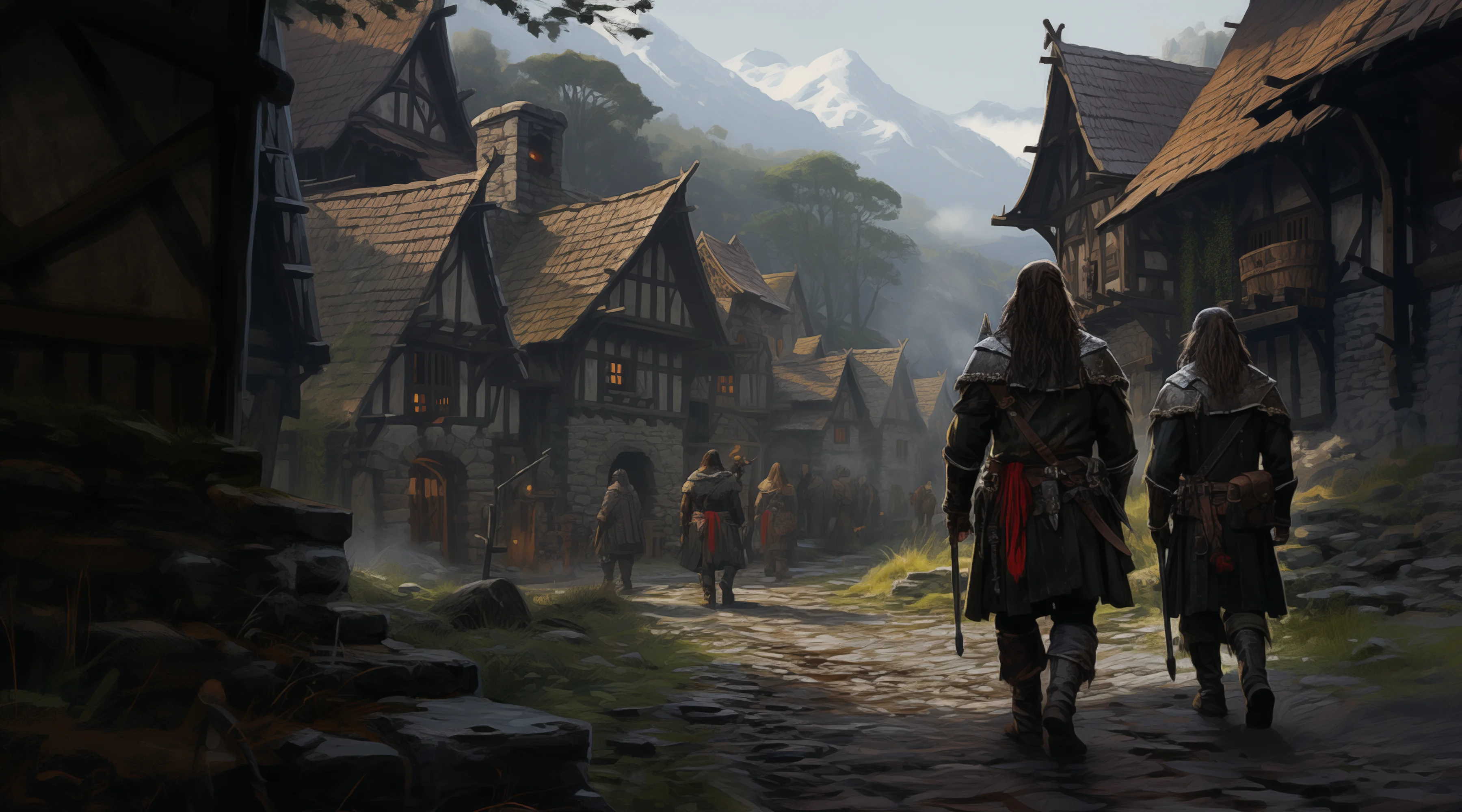
Standalone
Implementing Elliptic Curves
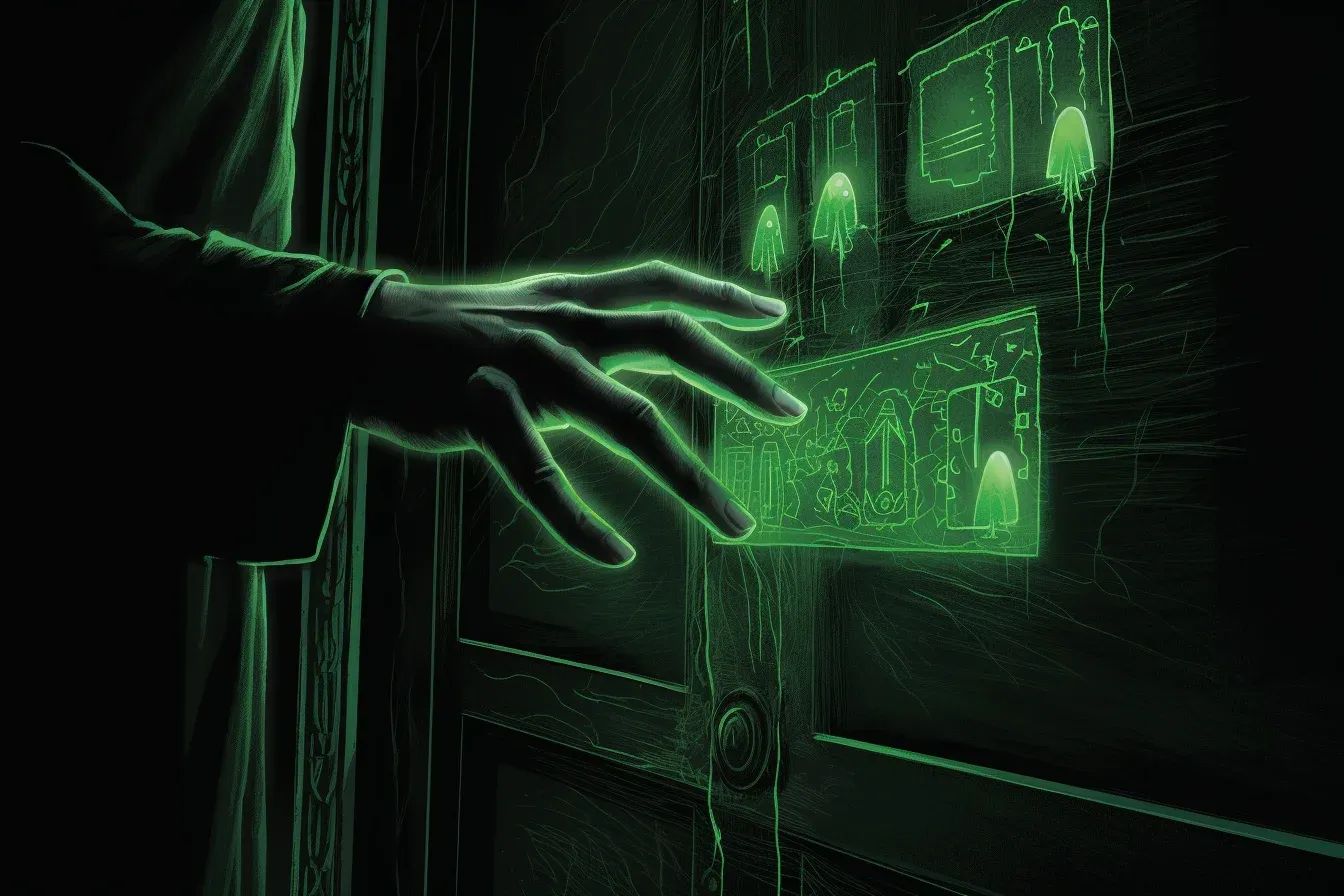
Part I
Field Arithmetic
Download quests in Questplay
Completing this quest requires knowledge in elliptic curve operations. If you are not read, we recommend trying our theory campaign first!
Take a look at contracts/ObsidianGates.sol
, a contract that uses an elliptic curve signature scheme for access control. The contract itself requires no modification, but for it to work, we must implement the underlying elliptic curve (in FieldElement.sol
and EllipticCurve.sol
).
ObsidianGates
uses the NIST P-256 curve, rather than the EVM's secp256k1 curve. The curve is given by the equation:
where:
The curve is restricted over a prime field , which uses modulo arithmetic for all field calculations. Hence, before implementing this curve in Solidity, it would be useful to first create a user-defined type for the field element.
In contracts/FieldElement.sol
, we have defined a new type Felt
(aka field element), along with some user-defined operators for +
, -
, *
, and ==
.
Felt is uint256;
using {
add as +,
sub as -,
mul as *,
equals as ==, inv
} for Felt global;
We want to define these operations to be modular operations under . By doing so, we can later comfortably handle field arithmetic when implementing the NIST P-256 curve itself.
Your Task
In contracts/FieldElement.sol
, implement the logic for add
, sub
, mul
, equals
, and inv
. These functions must be implemented efficiently.
You can safely assume that all Felt
inputs in functions will be within valid range (i.e., ).
Run tests in Questplay
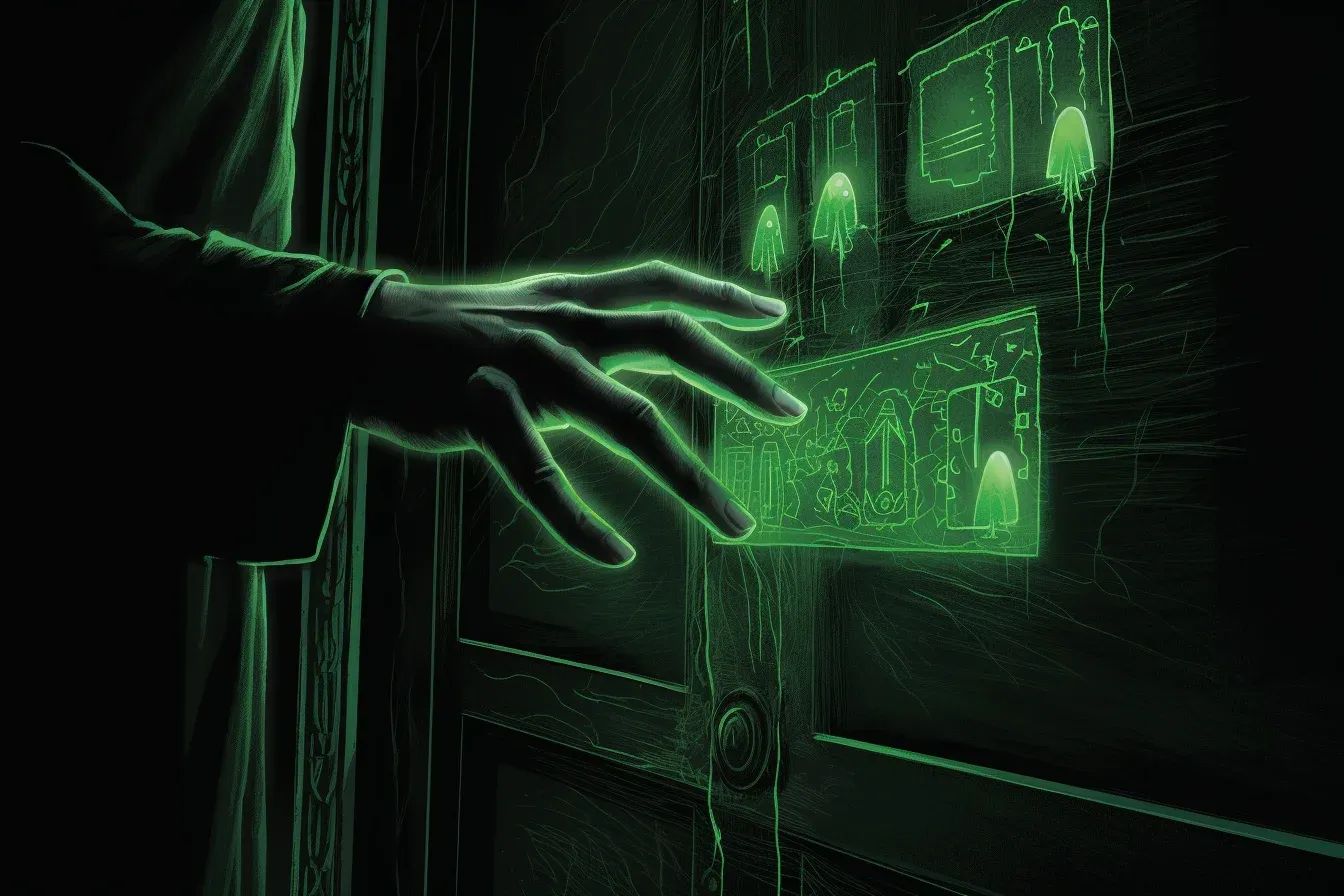
The Obsidian Gates are imbued with elliptic curve magic. To restore them, we must first build from the ground up...