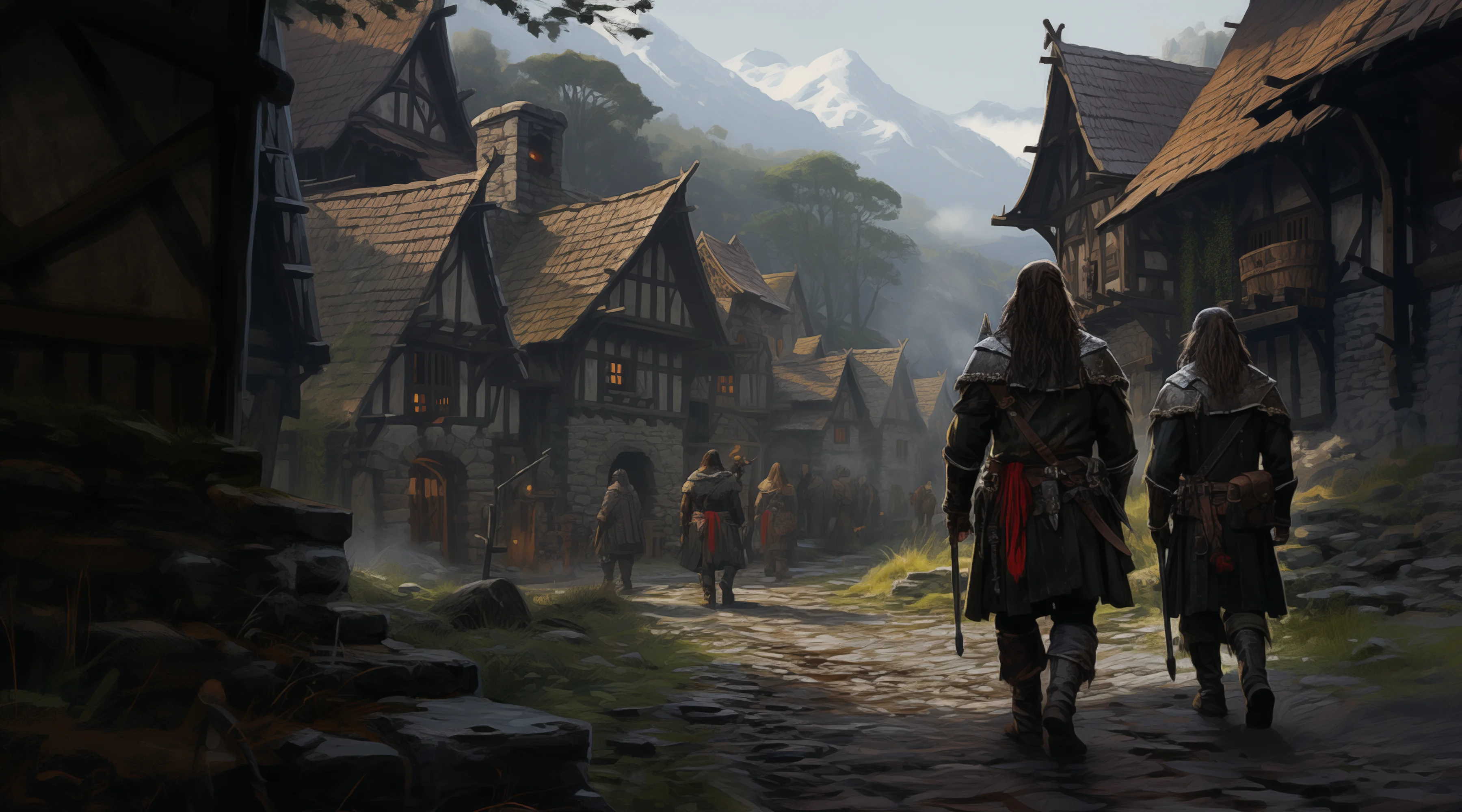
Standalone
Clone Factories
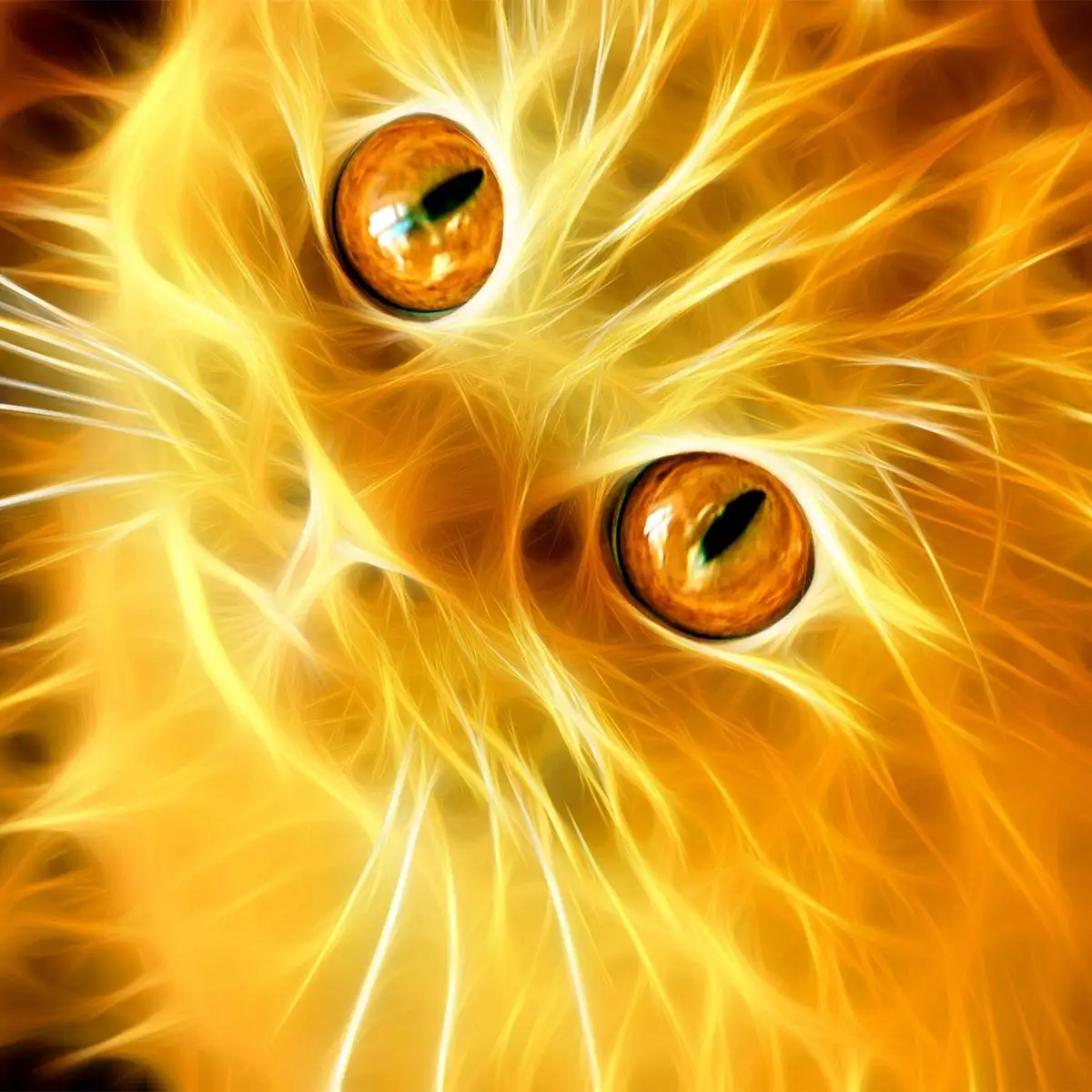
Part I
Factory Pattern
Download quests in Questplay
A SpiritCat
is a contract that holds onto ERC20 tokens for an owner.
The contract is designated to a recipient who can withdraw the tokens after a set amount of time.
if the recipient decides to withdraw the tokens early, they can only withdraw a partial amount. The rest of the tokens will then be permanently locked until the set amount of time has passed.
The amount of token the recipient can withdraw early is linearly correlated to time passed.
unlockedBalance=totalBalance*(timePassed/lockTime)
We would like to design an Elaine
contract that can deploy new SpiritCat
contracts.
ABI of Elaine
:
Events |
|
View Functions | Description |
| Returns the creator of the contract. |
Functions | Description |
| Creates a |
| Collects all remaining tokens from |
ABI of SpiritCat
:
View Functions | Description |
| Returns whether contract is active. |
| Recipient of tokens. |
| Returns |
| Address of ERC20 token. |
| Amount of ERC20 token not yet withdrawn. |
| Time when the recipient can fully withdraw tokens afterwards. |
| Amount of tokens the recipient can withdraw early. Should be |
Functions | Description |
| If |
| Can only be called by the contract's creator (i.e. |
Your Task
Complete the implementations in Elaine.sol
and SpiritCat.sol
.
You can assume that when summon()
is called, Summoner has ownership of sufficient amount of the corresponding ERC20 token.
Run tests in Questplay
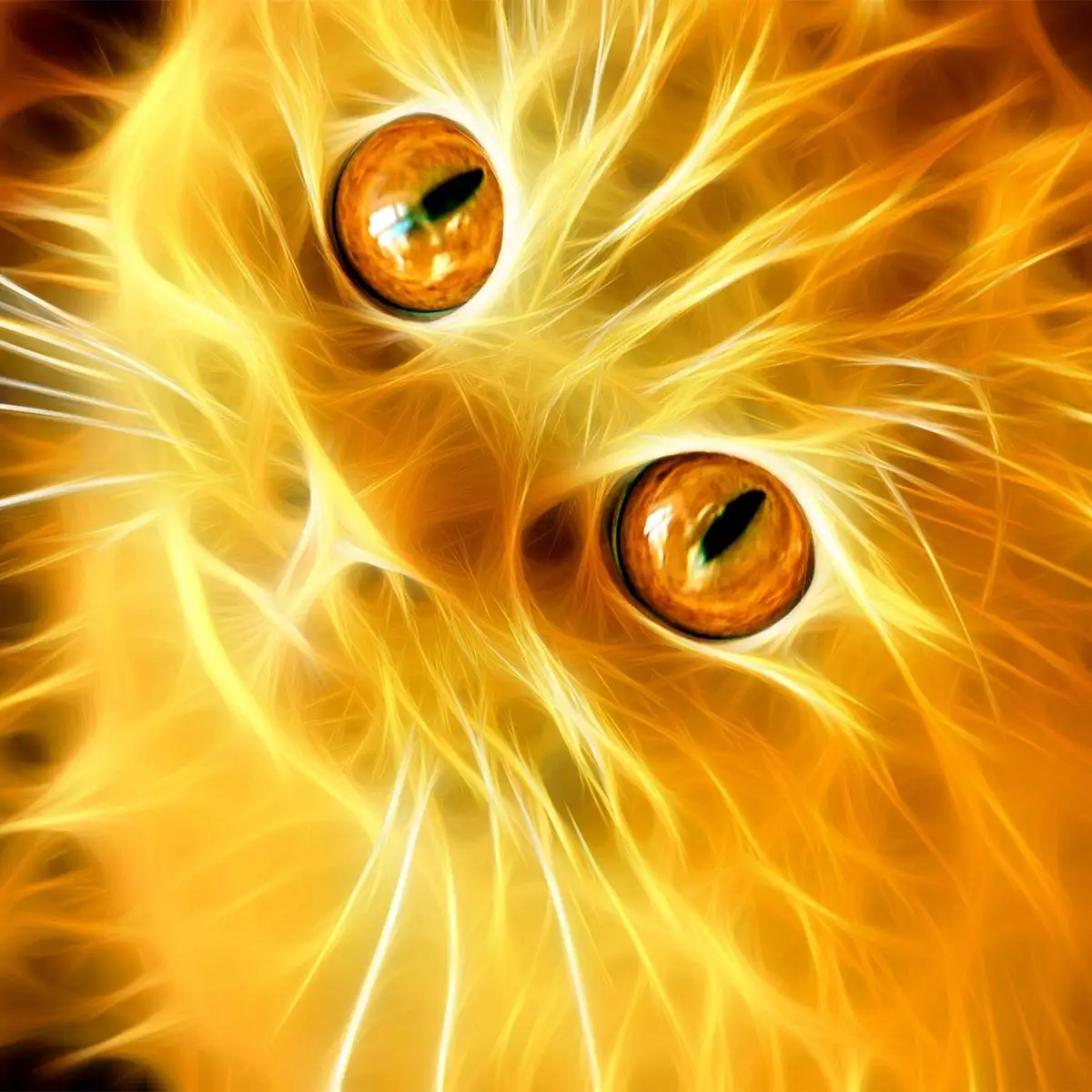
Spirit Cats are escrow spirits that hold onto coins for a recipient. They safekeep these coins, only returning them to their designated recipient slowly over time.