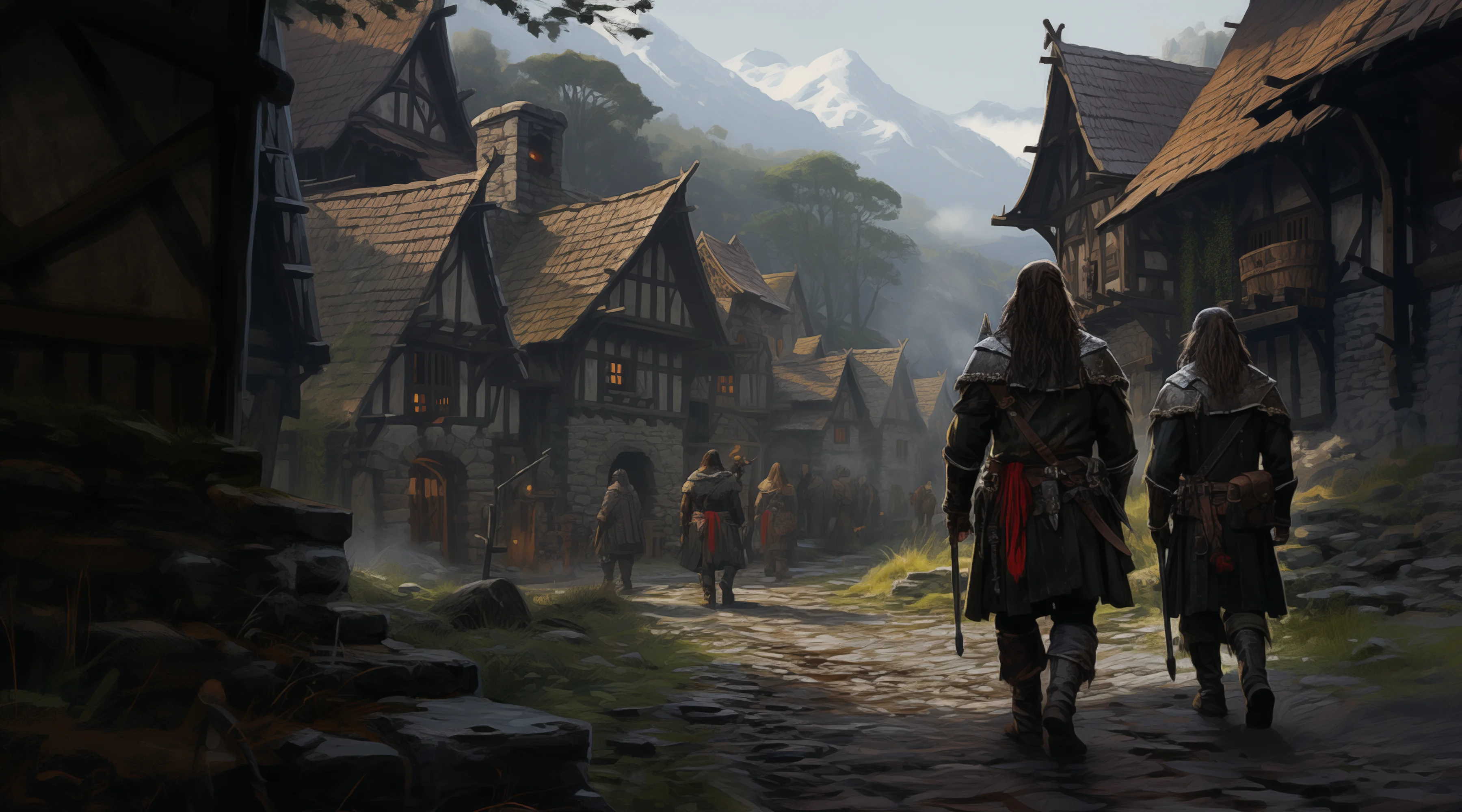
Standalone
Bypassing extcodesize()
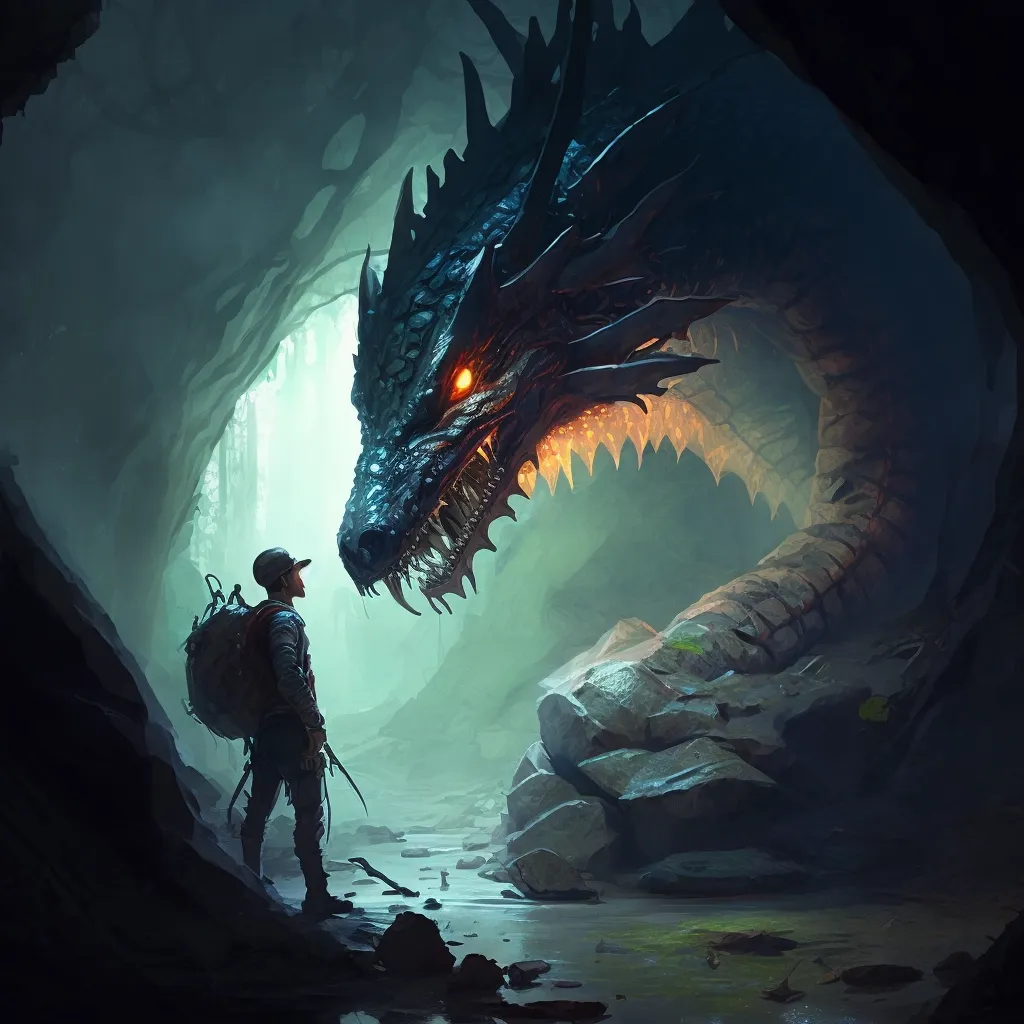
Verifying EOAs
Download quests in Questplay
View the contracts and any other additional content from your IDE.
The Basilisk hides in its den. For an address to challenge the Basilisk, it must implement the IChallenger
interface.
Unfortunately, the Basilisk plays dirty. It will only allow unarmed challengers into its den. Specifically, it does not allow smart contracts to enter.
Can you figure out how to beat the Basilisk?
Your Task
Set isSlain
in Basilisk
to true
.
Contract Code
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.19;
import "./interfaces/IChallenger.sol";
contract Basilisk {
mapping(address => bool) public entered;
bool public isSlain;
function _isContract(address account) private view returns (bool) {
uint256 size;
assembly {
size := extcodesize(account)
}
return size > 0;
}
function enter() external {
require(!_isContract(msg.sender), "The Basilisk detects a contract!");
// The Basilisk allows you to enter...
entered[msg.sender] = true;
}
function slay() external {
require(entered[msg.sender], "You have not entered the den!");
isSlain = IChallenger(msg.sender).challenge();
}
}
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.16;
interface IChallenger {
/// @notice Called by Basilisk. Should return true.
function challenge() external pure returns (bool);
}
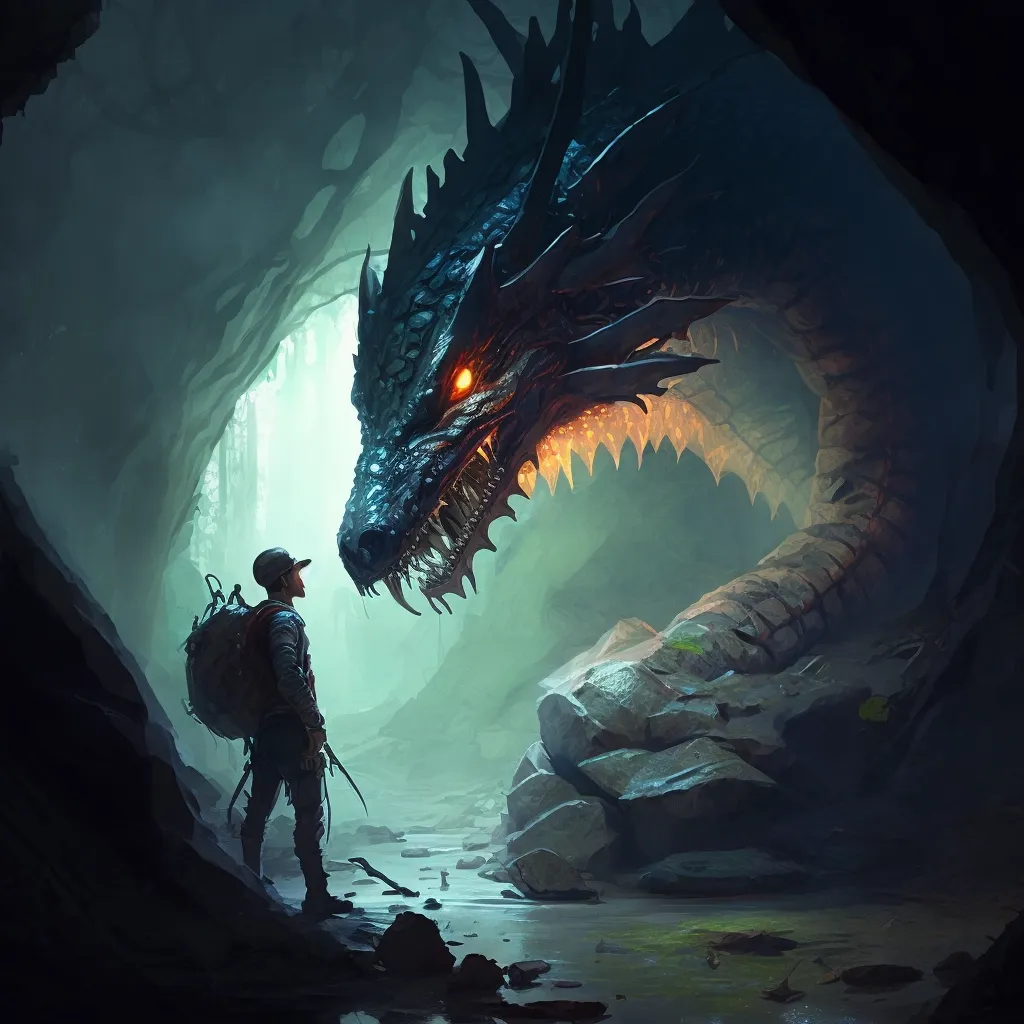
It’s time to enter the Basilisk’s den and slay the beast.